Most of the projects have routers. It is like a navigator of the application.
In this posting, we will learn about this feature on Vue3.
Create a Project
2021.06.02 - [Web/JavaScript] - [Vue3] Getting Started with Vue3 + TypeScript + TailwindCSS
Create a project to test.
And, add the router feature to the project.
$ vue add router
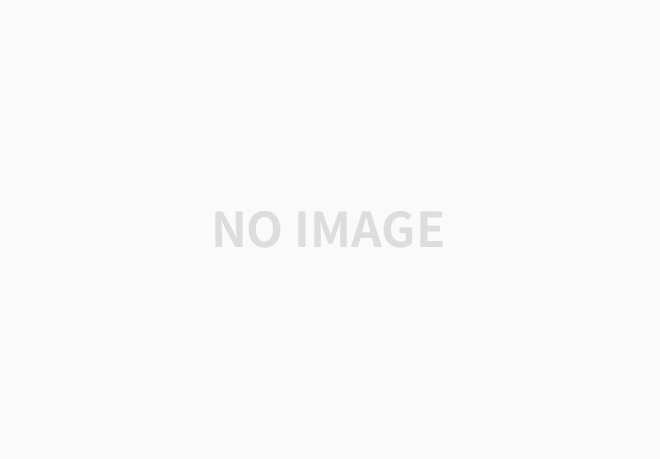
※ You can also create a project with a router through Vue-CLI.
Now, start the application.
$ yarn serve
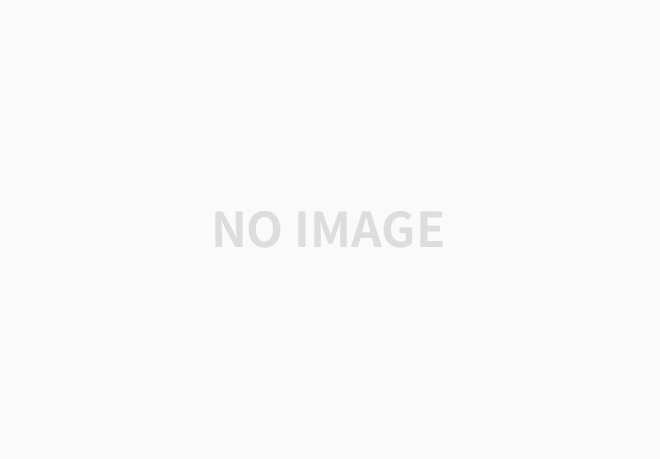
You can see the navigator from the top.
Look into the Application
Let's take a look at the router feature.
main.ts
import { createApp } from "vue";
import App from "./App.vue";
import "./assets/tailwind.css";
import router from "./router";
createApp(App).use(router).mount("#app");
Our application uses a router.
App.vue
<template>
<div id="nav">
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link>
</div>
<router-view />
</template>
This code creates a navigator and links components to it.
In the last, shows it to the user.
router/index.ts
import { createRouter, createWebHistory, RouteRecordRaw } from "vue-router";
import Home from "../views/Home.vue";
const routes: Array<RouteRecordRaw> = [
{
path: "/",
name: "Home",
component: Home,
},
{
path: "/about",
name: "About",
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () =>
import(/* webpackChunkName: "about" */ "../views/About.vue"),
},
];
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes,
});
This code defines routes as an array form and creates a router.
Making a router is pretty simple.
Add Our Own Path
Let's add our own route.
views/Hello.vue
<template>
<div class="flex flex-col justify-center items-center">
<h1>Hello!</h1>
<h2>This is an example route page.</h2>
</div>
</template>
App.vue
<template>
<div id="nav">
<router-link to="/">Home</router-link> |
<router-link to="/hello">Hello</router-link> |
<router-link to="/about">About</router-link>
</div>
<router-view />
</template>
router/index.ts
const routes: Array<RouteRecordRaw> = [
...
{
path: "/hello",
name: "Hello",
component: () =>
import(/* webpackChunkName: "hello" */ "../views/Hello.vue"),
},
...
];
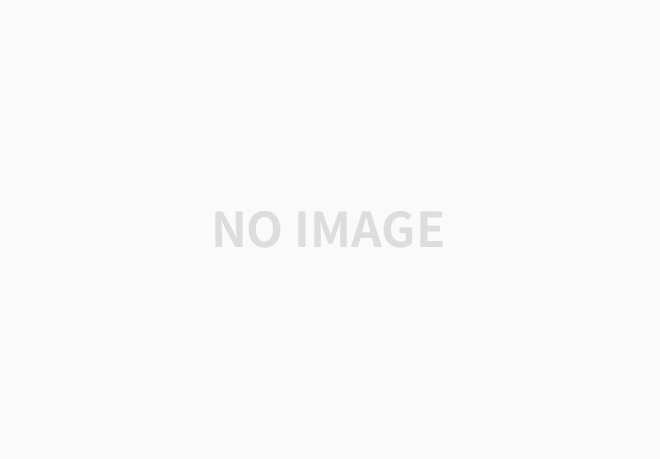
It works!! How easy to implement a router on Vue3.
Prefetch and Lazy Load
The prefetch feature was added From the Vue-CLI3.
The prefetch feature caches resources that can be used in the future, allowing users to quickly get resources when accessed.
Although it is a useful function, it makes to slow down the initial stage because all resources that will not use immediately are put in the cache.
Therefore, it should be used carefully.
For checking this feature, let's build our application.
$ yarn build
This is the status of current our application.
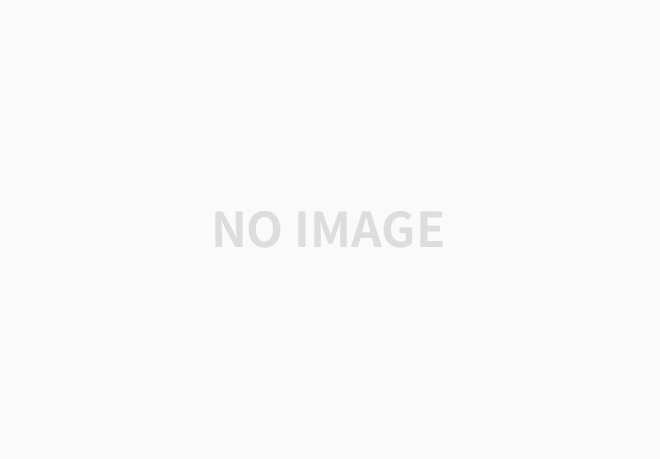
For comparison, let's disable the prefetch feature.
Create a configuration file for vue.
vue.config.js
module.exports = {
chainWebpack: (config) => {
config.plugins.delete("prefetch");
},
};
Now, build again.
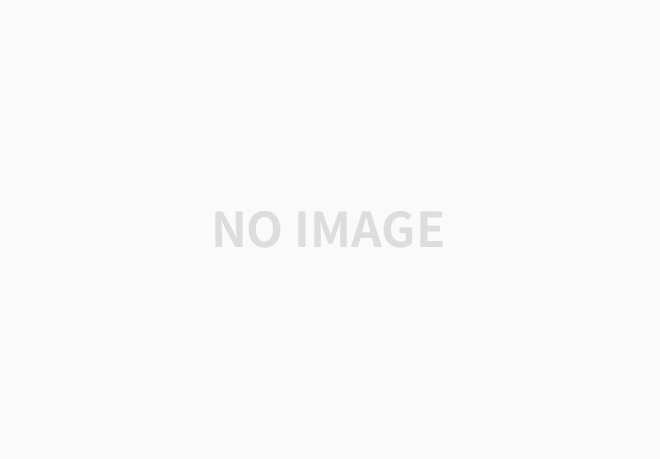
The loading is much fater than before, because only the necessary files are loaded.
However, resources that are not loaded are loaded at the time they are used, so it is slower at that time.
Please focus on the hello and about file.
These files are small in our application, but what if they were a larger application?
We can also use prefetch feature for specific files when it is disabled situation.
Let's apply prefetch feature to our Hello route.
router/index.ts
const routes: Array<RouteRecordRaw> = [
...
{
path: "/hello",
name: "Hello",
component: () =>
import(
/* webpackChunkName: "hello", webpackPrefetch: true */ "../views/Hello.vue"
),
},
...
];
Let's build and test it.
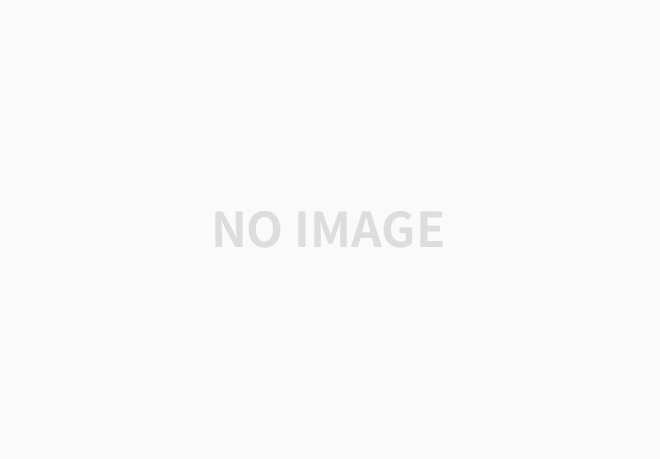
The hello file is loaded, but about file is not loaded.
Conclusion
We are now able to use router feature and know how to use prefetch feature.
'Web > JavaScript' 카테고리의 다른 글
[Vue3] Slot (v-slot) Usage (0) | 2021.06.20 |
---|---|
[Vue3] Component Usage (0) | 2021.06.20 |
[Vue3] Lifecycle (0) | 2021.06.16 |
[Vue3] Computed Property and Watcher (0) | 2021.06.12 |
[Vue3] Handling Loop using v-for (0) | 2021.06.09 |
댓글